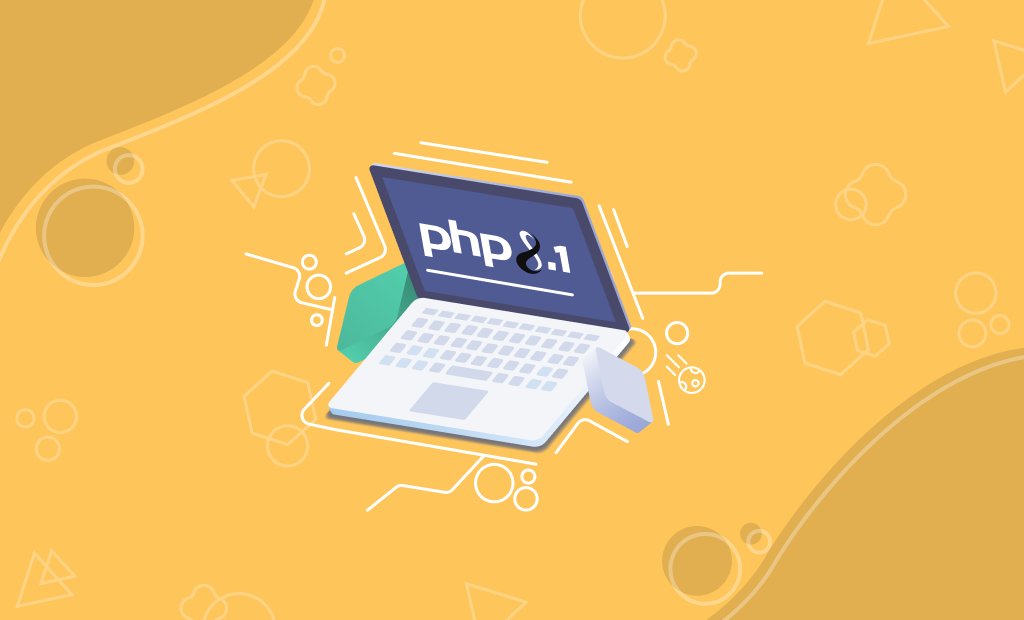
PHP 8.1 is Now Available at FastComet
Released for General Availability on November 25, 2021, PHP 8.1 is now fully available for all FastComet clients. We have performed our mandatory extensive testing and monitoring in order to ensure complete compatibility of the PHP 8.1 integration with cPanel and our infrastructure. We can state with confidence that it’s safe to deploy the new PHP version that provides stability and optimal performance for your PHP applications.
The new version of the programming language is packed with several exciting features. Those include pure intersection types, enums, fibers, new readonly
properties, and many more.
In this article, we’ll cover what’s new, changed, and deprecated in PHP 8.1—from its new features and performance improvements to significant changes and deprecations.
Table of Contents:
PHP 8.1 for all FastComet Users
PHP 8.1 is now available on all FastComet shared servers and all clients can easily select it via the FastComet PHP Selector. PHP 8.1 is the fastest version of PHP yet, which is why we recommend upgrading if your site is fully compatible with it. However, keep note that some plugins may not be compatible with the new version. Don’t forget to always check your website thoroughly after switching to a new PHP version.
New Features in PHP 8.1
Let’s start by covering the new features in PHP 8.1.
Pure Intersection Types
PHP 8.1 adds support for intersection types. It’s similar to union types introduced in PHP 8.0, but their intended usage is the exact opposite.
To understand its usage better, let’s refresh how type declarations work in PHP. Essentially, you can add type declarations to function arguments, return values, and class properties. This assignment is called type hinting and ensures that the value is of the correct type at call time. Otherwise, it throws up a TypeError right away. In turn, this helps you debug code better.
However, declaring a single type has its limitations. Union types help you overcome that by allowing you to declare a value with multiple types, and the input has to satisfy at least one of the declared types.
See how the RFC describes pure intersection types straight from the source.
Enums
PHP 8.1 is finally adding support for enums (also called enumerations or enumerated types). They’re a user-defined data type consisting of a set of possible values. The most common enums example in programming languages is the boolean type, with true and false as two possible values. It’s so common that it’s baked into many modern programming languages.
As per the enumerations RFC, enums in PHP will be restricted to “unit enumerations” at first.
The RFC delves further into enum methods, static methods, constants, constant expressions, and much more. Covering them all is beyond the scope of this article. You can refer to the documentation to familiarize yourself with all its goodness.
The Never Return Type
PHP 8.1 adds a new return type hint called never. It’s super helpful to use in functions that always throw or exit.
As per the RFC, URL redirect functions that always exit (explicitly or implicitly) are a good example for its use:
function redirect(string $uri): never {
header('Location: ' . $uri);
exit();
}
function redirectToLoginPage(): never {
redirect('/login');
}
A never-declared function should satisfy three conditions:
- It shouldn’t have the return statement defined explicitly.
- It shouldn’t have the return statement defined implicitly (e.g. if-else statements).
- It must end its execution with an exit statement (explicitly or implicitly).
The URL redirection example above shows both explicit and implicit usage of the never return type.
For further information on the never return type follow the official source.
Fibers
Historically, PHP code has almost always been synchronous code. The code execution halts till the result is returned, even for I/O operations. You can imagine why this process may make code execution slower.
There are multiple third-party solutions to overcome this obstacle to allow developers to write PHP code asynchronously, especially for concurrent I/O operations. Some popular examples include amphp, ReactPHP, and Guzzle.
However, there’s no standard way to handle such instances in PHP. Moreover, treating synchronous and asynchronous code in the same call stack leads to other problems.
Fibers are PHP’s way of handling parallelism via virtual threads (or green threads). It seeks to eliminate the difference between synchronous and asynchronous code by allowing PHP functions to interrupt without affecting the entire call stack.
Here’s what the RFC promises:
- Adding support for Fibers to PHP.
- Introducing a new Fiber class and the corresponding reflection class ReflectionFiber.
- Adding exception classes FiberError and FiberExit to represent errors.
- Fibers allow for transparent non-blocking I/O implementations of existing interfaces (PSR-7, Doctrine ORM, etc.). That’s because the placeholder (promise) object is eliminated. Instead, functions can declare the I/O result type instead of a placeholder object that cannot specify a resolution type because PHP does not support generics.
New readonly
Properties
PHP 8.1 adds support for readonly properties. They can only be initialized once from the scope where they’re declared. Once initialized, you cannot modify their value ever. Doing so would throw up an Error exception.
Its RFC reads:
A readonly property can only be initialized once, and only from the scope where it has been declared. Any other assignment or modification of the property will result in an Error exception.
Here’s an example of how you can use it:
class Test {
public readonly string $example;
public function __construct(string $example) {
// Legal initialization.
$this->example = $example;
}
}
Once initialized, there’s no going back. Having this feature baked into PHP greatly reduces boilerplate code that’s often used to enable this functionality.
The readonly
property offers a strong immutability guarantee, both inside and outside the class. It doesn’t matter what code runs in between. Calling a readonly
property will always return the same value.
However, using the readonly
property may not be ideal in specific use cases. For example, you can only use them alongside a typed property because declarations without a type are implicitly null and cannot be readonly
.
Furthermore, setting a readonly
property does not make objects immutable. The readonly
property will hold the same object, but that object itself can change.
Another minor issue with this property is that you cannot clone it. There’s already a workaround for this particular use case. Look into it if necessary.
A List of The Remaining PHP 8.1 New Features (with Links)
Because of the nature of this article, we cannot possibly go in detail about all the new features. That’s why we will give you a list of them with all the needed links to the official source:
- Define final Class Constants
- New fsync() and fdatasync() Functions
- New array_is_list() Function
- New Sodium XChaCha20 Functions
- New IntlDatePatternGenerator Class
- Support for AVIF Image Format
- New $_FILES: full_path Key for Directory Uploads
- Array Unpacking Support for String-Keyed Arrays
- Explicit Octal Numeral Notation
- MurmurHash3 and xxHash Hash Algorithms Support – MurmurHash3, xxHash, Algorithm-specific $options.
- DNS-over-HTTPS (DoH) Support
- File Uploads from Strings with CURLStringFile
- New MYSQLI_REFRESH_REPLICA Constant
- Performance Improvements with Inheritance Cache
- First-Class Callable Syntax
Changes in PHP 8.1
PHP 8.1 also includes changes to its existing syntax and features. We’ll go over some of them and list the rest in the same manner as with the new features.
PHP Interactive Shell Requires readline
Extension
PHP’s readline
extension enables interactive shell features such as navigation, autocompletion, editing, and more. While it’s bundled with PHP, it’s not enabled by default.
You can access the PHP interactive shell using PHP CLI’s -a command-line option:
php -a
Interactive shell
PHP >
php > echo "Hello";
Hello
php > function test() {
php { echo "Hello";
php { }
php > test();
Hello
Before PHP 8.1, you could open the interactive shell using PHP CLI even without the readline extension enabled. As expected, the shell’s interactive features didn’t work, rendering the -a option meaningless.
In PHP 8.1 CLI, the interactive shell exits with an error message if you’ve not enabled the readline extension.
php -a
Interactive shell (-a) requires the readline
extension.
MySQLi Default Error Mode Set to Exceptions
Before PHP 8.1, MySQLi defaulted to silent the errors. This behavior often led to code that didn’t follow strict Error/Exception handling. Developers had to implement their own explicit error handling functions.
PHP 8.1 changes this behavior by setting MySQLi’s default error reporting mode to throw an exception.
Fatal error: Uncaught mysqli_sql_exception: Connection refused in ...:...
As this is a breaking change, for PHP <8.1 versions, you should explicitly set the error handling mode using the mysqli_report function before making the first MySQLi connection. Alternatively, you can do the same by selecting the error reporting value by instantiating a mysqli_driver instance.
The RFC follows a similar change introduced in PHP 8.0.
Customizable Line Endings for CSV Writing Functions
Before PHP 8.1, PHP’s built-in CSV writing functions, fputcsv and SplFileObject::fputcsv, were hard-coded to add \n (or the Line-Feed character) at the end of every line.
PHP 8.1 adds support for a new parameter named eol to these functions. You can use it to pass a configurable end-of-line character. By default, it still uses the \n character. So, you can continue using it in your existing code.
Standard character escaping rules apply for using end-of-line characters. If you want to use \r, \n, or \r\n as EOL characters, you must enclose them in double-quotes.
Here’s the GitHub page tracking this new change.
A List of The Remaining PHP 8.1 Changes (with Links)
- New version_compare Operator Restrictions
- HTML Encoding and Decoding Functions Now Use ENT_QUOTES | ENT_SUBSTITUTE
- Warning on Illegal compact Function Calls
List of Deprecations in PHP 8.1
PHP 8.1 deprecates many of its previous features. The following list provides a brief overview of the functionalities PHP 8.1 deprecates:
- Can’t Pass null to Non-Nullable Internal Function Parameters
- Restricted $GLOBALS Usage
- Return Type Declarations for Internal Functions
- Serializable Interface Deprecated
- Non-Compatible float to int Conversions Deprecated
- The mysqli::get_client_info Method and mysqli_get_client_info($param) Deprecated
- All mhash*() Functions (hash Extension) Are Deprecated
- Deprecate autovivification on false
- The mysqli_driver->driver_version Property Is Deprecated
Other Minor Changes
There are many more deprecations in PHP 8.1. Listing them all here will be an exhausting exercise. We recommend you directly check the RFC for these minor deprecations. PHP’s GitHub page also includes a PHP 8.1 Upgrade Notes guide. It lists all the breaking changes you should consider before upgrading to PHP 8.1.
How to Upgrade to PHP 8.1
With FastComet’s shared hosting servers, you have the convenient option to select your PHP version quickly and easily. All you have to do is:
- Go to your to Client Area → cPanel → Select PHP Version:
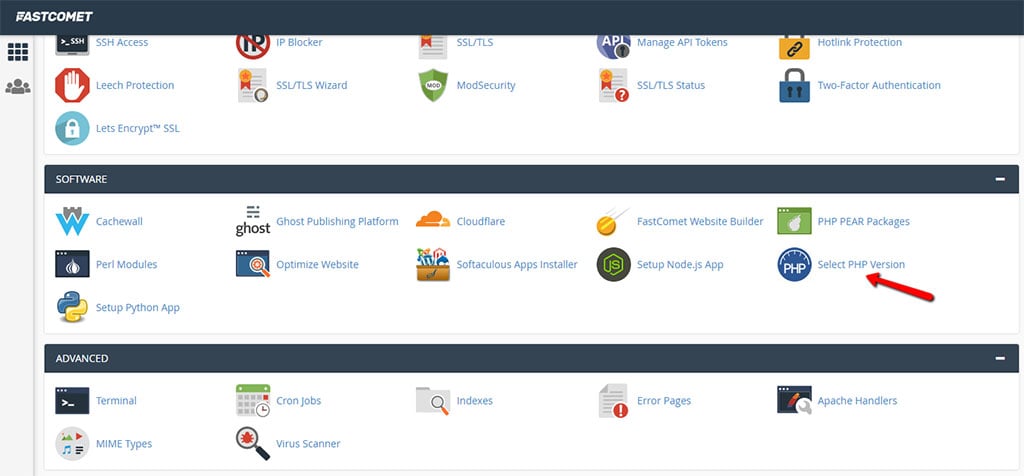
- In the PHP Selector, click on the drop-down:
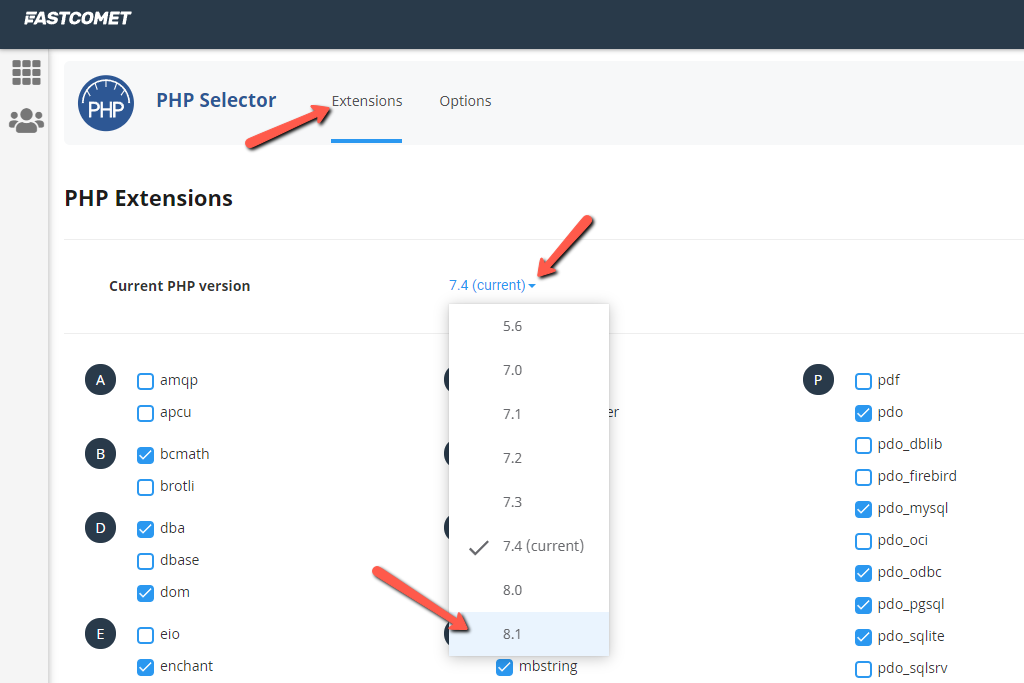
- Then, click on Set as Current:
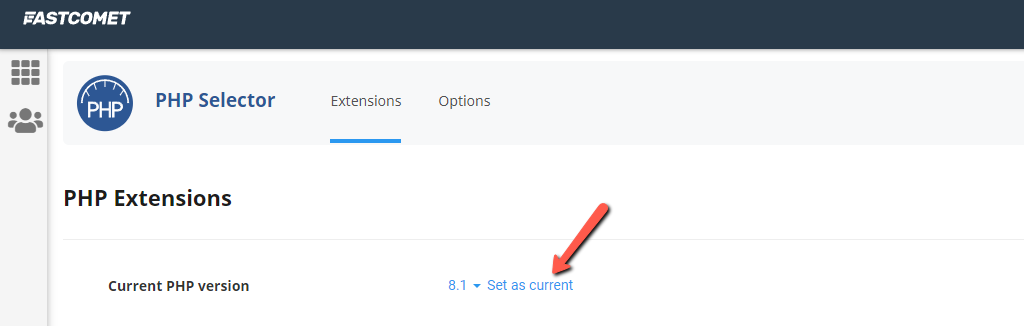
- Done!
When switching between different PHP versions, remember to check your project compatibility before beginning the transition.
Final Thoughts
Once again, we’ve covered all the key changes and improvements for the most recent PHP version—8.1. There are many changes this time around and we can’t wait to see what will come with the programming language’s new version.
Use the comment section to share with us which PHP 8.1 changes and improvements you like the most, or perhaps what you dislike. Happy Coding!
The latest tips and news from the industry straight to your inbox!
Join 30,000+ subscribers for exclusive access to our monthly newsletter with insider cloud, hosting and WordPress tips!
No Comments