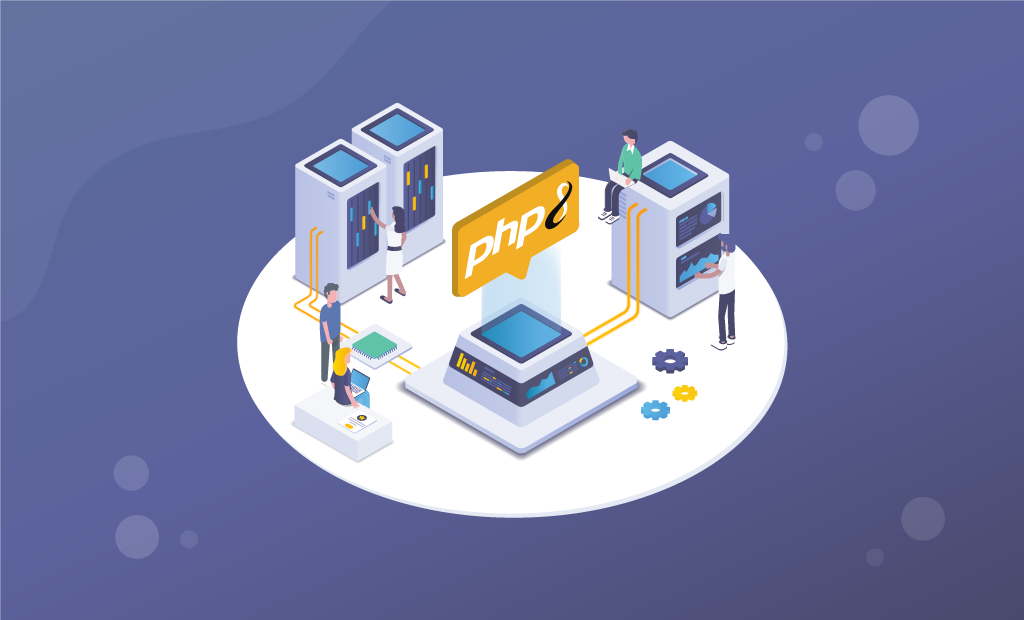
PHP 8.0 Deployed on FastComet (What’s New)
The major PHP 8 release is here on the FastComet servers. Although it was officially released for General Availability on November 26, 2020, it is now fully available for all FastComet customers. After our extensive testing and monitoring, to guarantee complete compatibility of the integration with cPanel and our infrastructure, we can confidently state that it is safe to deploy the version that provides stability and optimal performance for the deployed PHP applications.
This major update of the programming language brings many optimizations and powerful features. We’re excited to drive you through the most interesting changes to allow all programmers to write better code and build more powerful applications. As always, PHP 8 does not disappoint. This post covers some of the essentials around PHP 8 and how FastComet customers can benefit from the overall upgrade.
Table of Contents:
PHP 8.0 for all FastComet Users
PHP 8.0 is now available on all FastComet shared servers and can easily be selected via the FastComet PHP Selector. PHP 8.0 is the fastest version of PHP yet, so we recommend upgrading if your site is fully compatible with it. Naturally, keep in mind that some plugins may not be ready for the new version yet. That’s why you should check your website thoroughly after switching to PHP 8.0.
As we mentioned in our previous post regarding PHP 7.4, PHP 8.0 is a significant milestone. One of the reasons is the introduction to JIT — a management strategy that aligns raw-material orders from suppliers directly with production schedules. A program is being compiled on the fly into a form that’s usually faster, typically the host CPU’s native instruction set. To do this, the JIT compiler uses its access to dynamic runtime information. A standard compiler does not have the ability to perform this task.
PHP 7.4 is Now Selected as the Default PHP Version for New Accounts
From yesterday forward all new shared hosting accounts with us will be shipped with 7.4 by default. This upgrade will enhance the efficiency of the platform for hosting PHP websites, thanks to the benefits of 7.4. Of course, in case of any issues or incompatibilities, customers have the option to downgrade their version.
We are not yet setting PHP 8.0 as the default version on our shared hosting servers, mainly because there are still plugins, themes, etc., that may not be fully compatible with it.
Existing Accounts Should Best Perform the Switch Manually
All existing shared hosting account versions higher than 5.6 will remain unaffected and unchanged. Of course, for all of them, the upgrade to 7.4 or to 8.0 is just a click away.
We understand that managing the whole upgrade to a new version can be complex and may require your investment in the additional development effort. In order to provide you with enough time to ensure compatibility, we’ve implemented protective and preventative measures to extend PHP 5.6, 7.0, and 7.1 continuity on our platform past their December 2018 EOL date.
We advise all clients with Cloud VPS and Dedicated Server accounts to make the switch to the latest stable version of the scripting language.
Of course, you don’t have to install PHP 7.3 on your own. You can always request our expert Linux admins to perform the upgrade for you. They are available 24/7 for your convenience and will make sure your request is answered and processed swiftly.
What are the PHP 8.0 Improvements and Features?
Let’s start with all-new features.
Union Types
Given the dynamically typed nature of PHP, there are many cases where union types can be useful. Union types are a collection of two or more types that indicate you can use either one of those.
public function foo(Foo|Bar $input): int|float;
Note that void
can never be part of a union type since it indicates “no return value at all.” Furthermore, nullable
unions can be written using |null
, or by using the existing ?
notation:
public function foo(Foo|null $foo): void;
public function bar(?Bar $bar): void
Just in Time (JIT)
“JIT” stands for “just-in-time.” PHP is an interpreted language, meaning that it’s not compiled like a C, Java, or Rust program. Instead, it is translated to machine code — things that the CPU understands — at runtime.
“JIT” is a technique that will compile parts of the code at runtime so that the compiled version can be used instead.
Think of it as a “cached version” of the interpreted code generated at runtime.
There’s a “monitor” that will look at the code as it’s running. When this monitor detects parts of your code that are re-executed, it will mark those parts as “warm” or “hot,” depending on the frequency. Hot parts can be compiled as optimized machine code and used on the fly instead of the real code.
It’s enough to understand that a JIT compiler may significantly improve your program’s performance, but it’s a difficult thing to get right.
Knowing that the JIT compiler tries to identify hot parts of your code, you can see why it impacts the fractal example. There is a lot of the same calculations happening over and over again. However, since PHP is most often used in a web context, we should also measure the JIT’s impact there.
JIT opens the door for PHP to be used as a very performant language outside of the web. Additionally, it can be improved upon over time, which would also improve the code.
Named Arguments
Named arguments allow you to pass in values to a function by specifying the value name so that you don’t have to consider their order, and you can also skip optional parameters!
function foo(string $a, string $b, ?string $c = null, ?string $d = null)
{ /* … */ }
foo(
b: 'value b',
a: 'value a',
d: 'value d',
);
Attributes
Attributes, commonly known as annotations in other languages, offer a way to add metadata to classes without parsing docblocks.
As for a quick look, here’s an example of what attributes look like from the RFC:
use App\Attributes\ExampleAttribute;
#[ExampleAttribute]
class Foo
{
#[ExampleAttribute]
public const FOO = 'foo';
#[ExampleAttribute]
public $x;
#[ExampleAttribute]
public function foo(#[ExampleAttribute] $bar) { }
}
#[Attribute]
class ExampleAttribute
{
public $value;
public function __construct($value)
{
$this->value = $value;
}
}
Note that this base Attribute
used to be called PhpAttribute
in the original RFC but was changed with another RFC afterward.
Match Expression
You could call it the big brother of the switch expression: match can return values, doesn’t require break statements, can combine conditions, uses strict type comparisons, and doesn’t do any type of coercion.
It looks like this:
$result = match($input) {
0 => "hello",
'1', '2', '3' => "world",
};
Constructor Property Promotion
This RFC adds syntactic sugar to create value objects or data transfer objects. Instead of specifying class properties and a constructor for them, PHP can now combine them into one.
Instead of doing this:
class Money
{
public Currency $currency;
public int $amount;
public function __construct(
Currency $currency,
int $amount,
) {
$this->currency = $currency;
$this->amount = $amount;
}
}
You can now do this:
class Money
{
public function __construct(
public Currency $currency,
public int $amount,
) {}
}
New static
Return Type
While it was already possible to return self
, static
wasn’t a valid return type until PHP 8.0. Given PHP’s dynamically typed nature, it’s a feature that will be useful to many developers.
class Foo
{
public function test(): static
{
return new static();
}
}
Throw Expression
This RFC changes throw
from being a statement to being an expression, which makes it possible to throw exception in many new places:
$triggerError = fn () => throw new MyError(); $foo = $bar['offset'] ?? throw new OffsetDoesNotExist('offset');
Inheritance with Private Methods
Previously, PHP used to apply the same inheritance checks on public, protected, and private methods. In other words: private methods should follow the same method signature rules as protected and public methods. This doesn’t make sense since private methods won’t be accessible by child classes.
This RFC changed that behavior so that these inheritance checks are not performed on private methods anymore. Furthermore, the use of the final private function
also didn’t make sense, so doing so will now trigger a warning:
Warning: Private methods cannot be final as they are never overridden by other classes
Weak Maps
Built upon the weakrefs RFC added in PHP 7.4, a WeakMap
implementation is added in PHP 8.0. WeakMap
holds references to objects, which don’t prevent those objects from being garbage collected.
Take the example of ORMs. They often implement caches that hold references to entity classes to improve the performance of relations between entities. These entity objects can not be garbage collected as long as this cache has a reference to them, even if the cache is the only thing referencing them.
If this caching layer uses weak references and maps instead, PHP will garbage collect these objects when nothing else references them anymore. Especially in the case of ORMs, which can manage several hundred, if not thousands of entities within a request, weak maps can offer a better, more resource-friendly way of dealing with these objects.
Here’s what weak maps look like, an example from the RFC:
class Foo
{
private WeakMap $cache;
public function getSomethingWithCaching(object $obj): object
{
return $this->cache[$obj]
??= $this->computeSomethingExpensive($obj);
}
}
Allowing ::class
on Objects
A small, yet useful, new feature: it’s now possible to use ::class
on objects
, instead of having to use get_class()
on them. It works the same way as get_class()
.
$foo = new Foo();
var_dump($foo::class);
More Features
PHP 8.0 comes with a lot more new features, which we will list below:
- New
mixed
type; - Non-capturing catches;
- Trailing comma in
parameter
lists; - Create DateTime Objects from Interface;
- New stringable Interface;
- The
nullsafe
Operator - New
str_contains()
Function; - New
str_starts_with()
andstr_ends_with()
functions; - New
fdiv()
function; - New
get_debug_type()
Function; - New
get_resource_id()
Function; - Abstract methods in traits improvements;
- Object implementation of
token_get_all()
; - Type annotations for internal functions externals;
ext-json
always available.
You can visit the official source for some thorough information about the new major PHP update.
PHP 8.0 Overall Performance Benchmarks
With the major PHP 8.0 release, new features keep coming, and the programming language’s performance continues to evolve.
According to Phoronix benchmarks, PHP 8.0 continues to improve in terms of performance.
According to the benchmark stats, PHP 7+ shows improved memory usage, in addition to some other enhancements. As expected, PHP 8.0 is looking to be the fastest stable release yet.
Does your Application Support it?
The great news is that most web applications, including WordPress, fully work on PHP 8.0:
- WordPress 5.7;
- Joomla! 3.9.26;
- Laravel 6, 7, and 8;
- Symfony 5.2;
- CodeIgniter 4;
- CakePHP 4.1;
- PyroCMS 3.9;
- Bolt 3.7.2;
- ExpressionEngine 5.3.0.
As for Magento, the Magento community has begun its mission to make the Magento 2 PHP 8.0 compatible with the launch of the Magento PHP 8.0 Compatibility Community Project.
WordPress PHP 8.0 Usage Stats
With its release, WordPress 5.7 already had better compatibility with PHP 8.
Here is the percentage of PHP versions used with WordPress, as also shown in the pie chart below (according to WordPress.org):
- PHP 8.0: 0.3%;
- PHP 7.4: 27.3%;
- PHP 7.3: 29.1%;
- PHP 7.2: 18.4%;
- PHP 7.1: 4.5%
- PHP 7.0: 5.9%
- PHP 5.6: 9.8%;
- PHP 5.5: 1%;
- PHP 5.4: 1.6%;
- PHP 5.3: 1.4%;
- PHP 5.2: 0.6%;
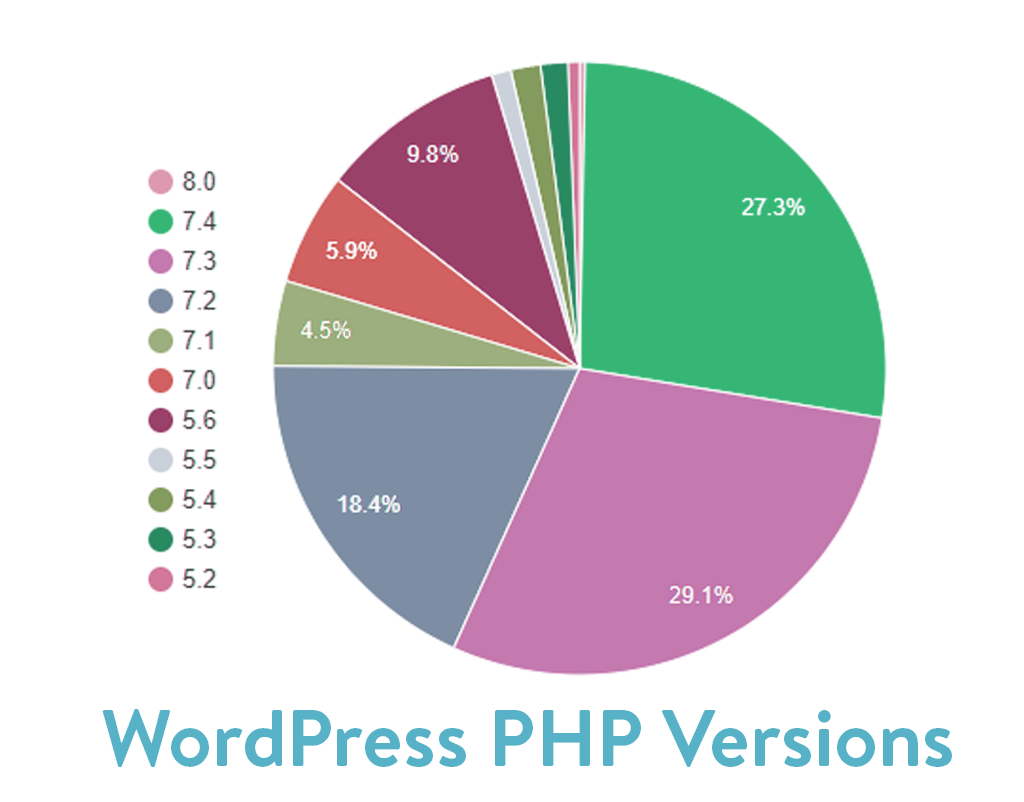
Unfortunately, PHP 5 is still used by 14.4% of all sites with a known server-side programming language. However, those stats are much better than a year ago, which could be due to the Site Health feature that reminds users to update their PHP version.
It’s encouraging that more than 50% of the WordPress websites are running on PHP 7.3 and above.
We recommend that you ask your host for a supported PHP version. Тhe best choice would be a release that is WordPress approved. As of the time this article is posted, the WordPress requirements are:
- PHP version 7.4 or greater;
- MySQL version 5.6 or greater or MariaDB version 10.1 or greater;
- HTTPS support;
How to Upgrade to PHP 8.0
With FastComet’s shared hosting servers, you have the convenient option to select your PHP version quickly and easily. All you have to do is:
- Go to your to Client Area → cPanel → Select PHP Version:
- In the PHP Selector, click on the drop-down:
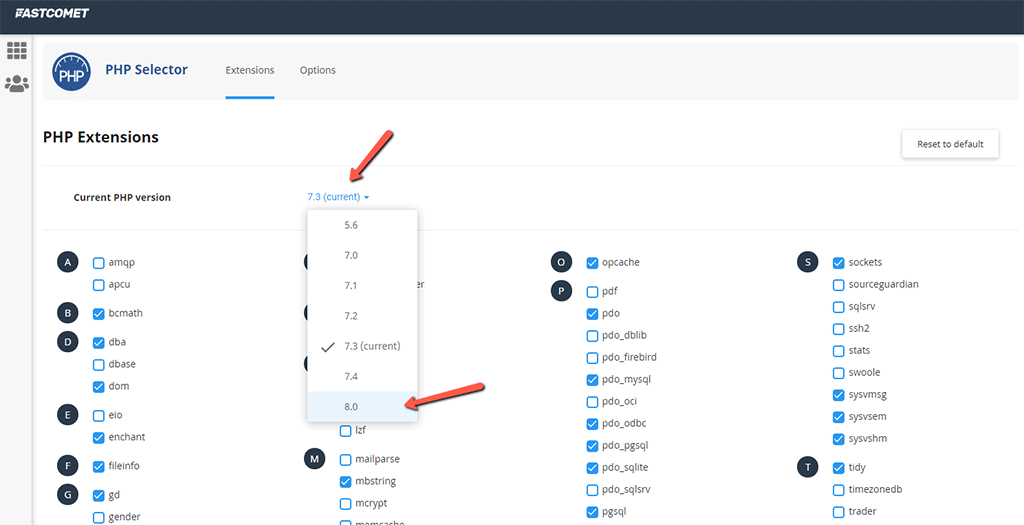
- Then, click on Set as Current:
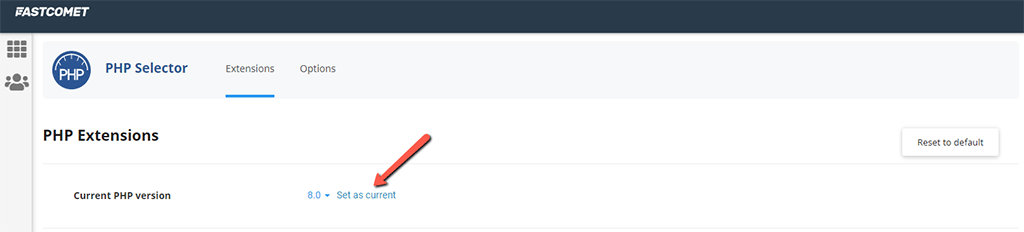
- Done!
When switching between different PHP versions, remember to check your project compatibility before beginning the transition.
Final Words
We’ve covered all the PHP 8.0 key changes and improvements. The most significant addition is surely the JIT compiler, but that doesn’t mean everything else that comes with this major PHP version is not important.
Feel free to share in the comments which of the PHP 8.0 changes and improvements you like the most, or perhaps what you dislike. Happy Coding!
The latest tips and news from the industry straight to your inbox!
Join 30,000+ subscribers for exclusive access to our monthly newsletter with insider cloud, hosting and WordPress tips!
No Comments