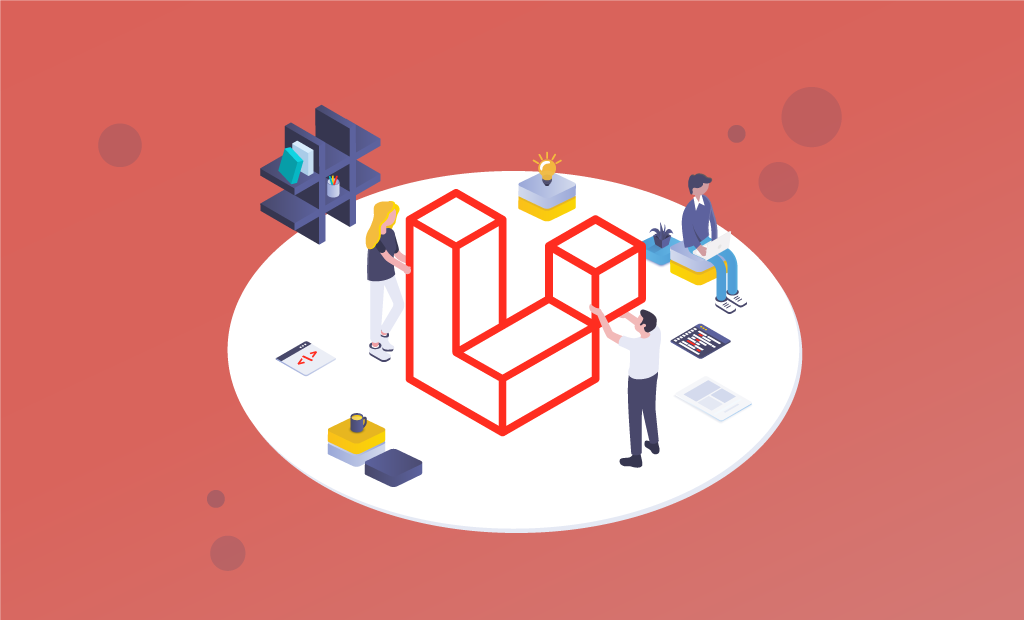
Laravel 6.0 Comes in Hot with LTS
Yes! Laravel 6.0 is officially out now! Since you are here, you probably already know that Laravel is one of the most popular PHP frameworks, used by developers across the globe. That means we are all on the same hype-train and we want to find out what the new LTS Laravel 6.0 version has to offer. We are all in for a treat with this release, because it’s as good as it can be. So, without any further ado, let’s get to it.
This post will cover:
A Quick Preview of Laravel 6.0
The new Laravel version, 6.0, continues with the improvements that were made in Laravel 5.8. Laravel 6.0 (LTS*) introduces semantic versioning, Laravel Vapor compatibility, job middleware, improved authorization responses, lazy collections, a new error page, sub-query improvements, a variety of bug fixes and usability enhancements, and the extraction of frontend scaffolding to the laravel/ui
Composer package.
*LTS – Long Term Support. For LTS releases like Laravel 6.0, we get bug fixes for 2 years, with security fixes being provided for 3 years. LTS releases offer the most extended period of support and maintenance. Meanwhile, for general releases (non-LTS), bug fixes are provided for 6 months and security fixes for 1 year. For additional libraries like Lumen, only the most recent version gets bug fixes.
Semantic Versioning
Currently, Laravel is following the semantic versioning standard, making the framework consistent with all other first-party Laravel packages that already followed the same versioning standard. The current 6-months Laravel release cycle will remain unchanged.
Laravel Vapor Compatibility
Laravel 6.0 comes with Laravel Vapor compatibility, which is an auto-scaling serverless* deployment platform. Vapor is abstracting the whole complexity of all managing Laravel applications on AWS Lambda. Additionally, Laravel Vapor interfaces those applications with databases, SQS queues, networks, Redis clusters, CloudFront CDN, and more.
*Serverless – examples for serverless are Google Cloud Functions, Amazon Lambda, etc. You can deploy to their platform without thinking about infrastructure. There are servers, of course, but you never have to worry about them. You probably used Horizon when managing your queues. When serverless, you can forget about it. Even if you get a thousand jobs on your queue, they will be executed in a manner of seconds. The whole thing is elastically scaled, and if nobody is using your app, you will not be getting charged for it.
What Vapor Offers
- On-demand auto-scaling with no need for server maintenance. You can watch your web application, and workers scale in a matter of seconds – all on demand.
- Databases. Vapor is not only about deployments. You can create, scale, manage, and even restore traditional or serverless databases from the Vapor Dashboard.
- Caches. If you need data fast, Vapor will make it easy to create and manage ElastiCache Redis clusters. If you need speed, Vapor scales your cache without you having to lift a finger.
- A Serverless Laravel Future. Laravel Vapor is fine-tuned by Laravel’s creators in order to work seamlessly with the framework. You will be able to keep writing your Laravel applications in the same way that you’re used to writing them.
- Queues. You can write and dispatch Laravel jobs. You will be able to experience the power of serverless scalability with hundreds of jobs being executed concurrently without the need for any configuration.
- Cloud Storage. You can stream your file uploads easily and directly to S3 with the built-in JavaScript utilities that Vapor has.
Job Middleware
Job middleware allows for wrapping custom logic around the execution of queued jobs, which serves to reduce the jobs boilerplate. For instance, in some of the previous Laravel versions, you may have wrapped the logic of a job’s handle
method within a rate-limited callback:
/**
* Execute the job.
*
* @return void
*/
public function handle()
{
Redis::throttle('key')->block(0)->allow(1)->every(5)->then(function () {
info('Lock obtained...');
// Handle job...
}, function () {
// Could not obtain lock...
return $this->release(5);
});
}
In version 6.0, this logic can be extracted into a job middleware, which would allow you to keep your job’s handle
method free of all rate-limiting responsibilities:
< ?php
namespace App\Jobs\Middleware;
use Illuminate\Support\Facades\Redis;
class RateLimited
{
/**
* Process the queued job.
*
* @param mixed $job
* @param callable $next
* @return mixed
*/
public function handle($job, $next)
{
Redis::throttle('key')
->block(0)->allow(1)->every(5)
->then(function () use ($job, $next) {
// Lock obtained...
$next($job);
}, function () use ($job) {
// Could not obtain lock...
$job->release(5);
});
}
}
Following the creation of middleware, they can be attached to a job by returning them from the job middleware
method:
use App\Jobs\Middleware\RateLimited;
/**
* Get the middleware the job should pass through.
*
* @return array
*/
public function middleware()
{
return [new RateLimited];
}
Improved Authorization Responses
With previous Laravel releases, it was not easy to acquire and expose the custom authorization messages to the end-users. That’s what made it hard to explain to end-users why a certain request has been denied. In Laravel 6.0, the procedure is much easier via authorization response messages and the brand new Gate::inspect
method. Here’s an example, given the policy method:
/**
* Determine if the user can view the given flight.
*
* @param \App\User $user
* @param \App\Flight $flight
* @return mixed
*/
public function view(User $user, Flight $flight)
{
return $this->deny('Explanation of denial.');
}
The response and the message of the authorization policy can be retrieved via the Gate::inspect
method with ease:
$response = Gate::inspect('view', $flight);
if ($response->allowed()) {
// User is authorized to view the flight...
}
if ($response->denied()) {
echo $response->message();
}
Additionally, the custom messages automatically return to the frontend, using helper methods such as $this->authorize
or Gate::authorize
from the routes or the controllers.
Lazy Collections
There are plenty of developers that are already enjoying Laravel’s robust Collection methods. To further improve the powerful Collection
class, Laravel 6.0 is introducing LazyCollection
. It leverages PHP generators, allowing you to work with extensive datasets while at the same time keeping your memory usage as low as possible.
Imagine that your application has to process a multi-gigabyte log file while taking advantage of Laravel’s collection
methods to parse the logs. Instead of reading the whole file into memory at once, lazy collections can be used for keeping only a certain small part of the file in memory at a given time:
use App\LogEntry;
use Illuminate\Support\LazyCollection;
LazyCollection::make(function () {
$handle = fopen('log.txt', 'r');
while (($line = fgets($handle)) !== false) {
yield $line;
}
})
->chunk(4)
->map(function ($lines) {
return LogEntry::fromLines($lines);
})
->each(function (LogEntry $logEntry) {
// Process the log entry...
});
Or, let’s say you have to iterate through 10,000 Eloquent models. When you use traditional Laravel collections, all of the 10,000 Eloquent models need to be loaded into memory simultaneously:
$users = App\User::all()->filter(function ($user) {
return $user->id > 500;
});
With all that said, starting in Laravel 6.0, the query builder’s cursor
method gets an update and returns a LazyCollection
instance. That allows users to run only a single query against the database, but at the same time only keep a single Eloquent model at a time, loaded in memory. In the following example, the filter
callback isn’t executed until you iterate over every single user, which allows for a drastic reduction in memory usage:
$users = App\User::cursor()->filter(function ($user) {
return $user->id > 500;
});
foreach ($users as $user) {
echo $user->id;
}
Eloquent Subquery Enhancements
Laravel 6.0 is introducing some new improvements to database subquery support. Let’s use the Laravel example and imagine we have two tables – one of flight destinations
and another of flights
to destinations. The flights
table has an arrived_at
column that indicates the flight’s arrival at its destination.
Using the new subquery select functionality in Laravel 6.0, we can select all of the destinations
and the name of the flight that most recently arrived at that destination using a single query:
return Destination::addSelect(['last_flight' => Flight::select('name')
->whereColumn('destination_id', 'destinations.id')
->orderBy('arrived_at', 'desc')
->limit(1)
])->get();
In addition, we can use new subquery features added to the query builder’s orderBy
function to sort all destinations based on when the last flight arrived at that destination. Again, it can all be done while executing a single query against the database:
return Destination::orderByDesc(
Flight::select('arrived_at')
->whereColumn('destination_id', 'destinations.id')
->orderBy('arrived_at', 'desc')
->limit(1)
)->get();
Laravel UI
The frontend scaffolding provided with previous Laravel versions has been extracted into a laravel/ui
Composer package. That allows the first-party UI scaffolding to be developed and versioned separately from the primary framework. As a result, there is no present Bootstrap or Vue code present in default framework scaffolding, and the make:auth
command has been extracted from the framework too.
To restore the traditional Vue/Bootstrap scaffolding that is present in previous Laravel releases, you should install the laravel/ui
package and use the ui
Artisan command to install the frontend scaffolding:
composer require laravel/ui
php artisan ui vue --auth
Ignition – the New Error Page for Laravel
Last, but not least! With version 6.0, Ignition becomes the Laravel error page. The open source package was presented at LaraconEU by Freek Van der Herten and Marcel Pociot. Ignition has some attractive Laravel-specific features which should make debugging exceptions and stack traces much, much better. One might even enjoy throwing an exception on purpose to see this beautiful new debugging stack for Laravel.
In ignition, there are several tabs with helpful info about the request, the app/framework, user, context, and debug. The debug tab has a neat feature to show queries, logs, and dumps. When a dump happens, Ignition will display the filename where the statement occurred. You don’t have to search for the missing dd()
call in a vendor package, not anymore!
Apart from everything else, Ignition even goes the extra mile and makes suggestions when common problems occur. Keep in mind that Ignition is the default error page for Laravel 6.0, but it’s also installable with existing Laravel 5 apps.
Final Thoughts
As you can see for yourself, the long-awaited LTS Laravel 6.0 is as good as it can be. Are you using Laravel 5.8 and want to jump right on the newest version? FastComet strongly suggests creating a backup manually, even if we do them for you daily. Also, don’t forget that Laravel 6.0 requires at least PHP 7.2. Luckily, with FastComet you already have PHP 7.2 Enabled by Default. If you need thorough instructions on installing Laravel 6.0, follow their official Upgrade Guide.
If you enjoyed this article, then you’ll love FastComet’s Laravel SSD Cloud hosting platform. Experience increased power, flexibility, and control for your applications.
While we can go on and on with how awesome the new Laravel version is, there is actually no need for that. We have covered the most important features that came with the new release, and we are more than happy about them. You are welcome in the comment section to share your thoughts about Laravel 6.0. Which features or improvements do you like the most and why? And as always, we wish you Happy Coding!
The latest tips and news from the industry straight to your inbox!
Join 30,000+ subscribers for exclusive access to our monthly newsletter with insider cloud, hosting and WordPress tips!
No Comments